NodeJS Toolkit
This quick guide will guide you on how to use the NodeJS toolkit to quickly develop microservices.
Getting Start
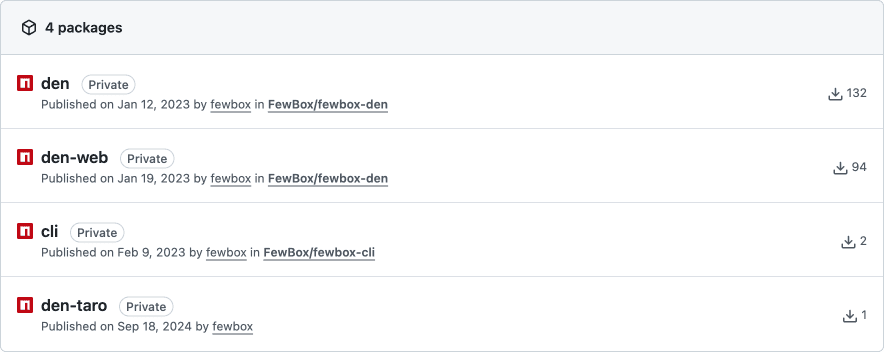
Install
cli
1yarn global add cli
app
1yarn add den
web
1yarn add den-web
wechat
1yarn add den-taro
Uninstall
cli
1yarn global remove cli
app
1yarn remove den
web
1yarn remove den-web
wechat
1yarn remove den-taro
Usage
yarn
1yarn add sass -D
2yarn add @paypal/react-paypal-js
3yarn add jwt-decode
4yarn add react-ga4
5yarn add react-intl
App.tsx & /style/_variables.scss
1import '../style/_root.scss';
2//Change the variables. (EG. $body-color: #e1e1e1;)
example
1import { Den } from @fewbox/den'; // @fewbox/den-web @fewbox/den-taro @fewbox/den-lite
2
3<Den.Components.VLabel frontColor={Den.Components.ColorType.Primary} caption=Hello, FewBox!' />
Class Component
1import * as React from 'react';
2import { Den } from @fewbox/den';
3import './index.scss';
4
5export interface IClassComponentProps extends Den.Interface.IBaseProps {
6 message: string;
7}
8
9export interface IClassComponentStates extends Den.Interface.IBaseStates {
10}
11
12export default class ClassComponent extends Den.Components.BaseComponent<IClassComponentProps, IClassComponentStates> {
13 render(): React.ReactNode {
14 return <Den.Components.VBoundary {...this.props} className={this.getClassName('class-component')} style={this.getStyle()}>
15 <Den.Components.VLabel caption={this.props.message} />
16 </Den.Components.VBoundary>;
17 }
18}
Function Component
1import * as React from 'react';
2import { Den } from @fewbox/den';
3import './index.scss';
4export interface IFunctionComponentProps extends Den.Interface.IBaseProps {
5 isSelected: boolean;
6}
7export interface IFunctionComponentStates extends Den.Interface.IBaseStates {
8 isSelected: boolean;
9}
10const FunctionComponent = (props: IFunctionComponentProps) => {
11 const _base = Den.Components.Base(props);
12 const [state, setState] = React.useState<IFunctionComponentStates>({ isSelected: props.isSelected });
13 return (
14 <div className={_base.getClassName(`function-component${state.isSelected ? ' selected' : ''}`)} style={_base.getStyle()} onClick={() => { setState({ ...state, isSelected: !state.isSelected }); }}>
15 Click Me
16 </div>
17 );
18}
19export default FunctionComponent;